???Java??????????Python??????
???????????? ???????[ 2017/7/4 10:28:57 ] ??????????????????? Java Python
????def log_calls(old_function):
????def new_function(*args?? **kwargs):
????print("i'm being called!"?? args?? kwargs)
????return old_function(*args?? **kwargs)
????return new_function
????@log_calls
????def foo(a?? b?? c=3):
????print(f"a = {a}?? b = ?? c = {c}")
????foo(1?? b=2)
????# i'm being called! (1??) {'b': 2}
????# a = 1?? b = 2?? c = 3
???????е????????????? ?????????????????ι?????; ??????foo?????new_function?滻?????????е?????????foo?? foo??????????????????Щ????????Щ?????
??????????????ж????? ?????????????????????????????棬?????????????? ??????????????????????????????????????????????????????????
????????????????
???????????????????????????????????????? — ?????????????????С??? C ???? C++ ????????????????ж???????;???????????“?決”??????????????????????????????????????????????????棬Java??????ж???????!?????????????????????????????
????Python ?????з????????????????????????????????????????????????????????????????????????á?
?????? Python ??????????? ?????ж????????????Python ??????????????????????????塣 ????????????????????????? Python???????????? — ????????????????????????????????
????????????????????? pytest?? ????????????? Python ?? assert ?????? ????? assert x == 1 ? false ????????????? AssertionError ?????????????????????????????????????????? Python ?????????????? — ?? JUnit ???????????????? — ????????????????????? assertEquals????????????Щ?????????ò???????????????????????????????? pytest ?У? assert x == 1 ??????????????pytest ???????? x ??… ?????????б??????з??磬??????????????Щ??????? ??????????????е???Щ???????????????????????????????????
????pytest????ι???????? ??????????????????????????pytestд???? — ??????????
?????????????????????????????? ????????????????????Щ????????????????Щ?????????????????????Щ?????????????????????С? ???? Python ???????????????????????????????????? — ??Щ?????????????????????
????????
????????(??C#????????????)????????????????????????? Java ?У???? Point ?????????? getX() ?? setX() ??????????????????? x ????????????????????? x ?????д???????????????? Python ?У????????????????Щ?? ??????????????????????
class Point:
????def __init__(self?? x?? y):
????self._x = x
????self._y = y
????@property
????def x(self):
????return self._x
????# ... same for y ...
????point = Point(3?? 4)
????print(point.x) # 3
????????? @property ??????????Σ??????? Java ??????????????????????????????????????????????——??????????????????——?????????????????????????????????? Java ???????????????? API ???????????????????塣(??????????? x ????????????????????д????!??д????????е????????????????????ι???????????????漲??????????????? point.x??)
??????????????????????????????У????? C#???????????????????????????? Python ?????????????????????????????????????????????????δ?????????????? Python ???????????????? Python ???????????????????????????????????????????????????????????????????з??????????????????????????????????????
????????????????????????????????????????????????????——?????????????????????? Python ??????
??????????????????????????? public ?? private ???η?????????Python ?в???????????????????????????????????“???”——?????????????“??????????????? API ???????”???????????????????壬Phthon ???????????????????????????(????????????????????)???????????final ??static??const??
???????????????????????? Python ???????????????κ????顣???????????????????á????????????????????д???????????????з????????????????? BUG???????????????????????????????????????????????????????????? BUG??????????????????????????
????????????????????д????????????????——???統(tǒng)???????????????У?????????????? time.time() ?滻??????????????????????????(?? Java ???)?????? Python ???????????????????????????????????????????????鵽??????
??????
????Java ?????? Class ???????????????????????????????? Foo ??? Class ?????? Foo.class???????? Foo ?????????????????????????????????Java ????????????????????Щ????????
????Python ?У??????????????????(??????????????????????????????????????)????????????????????????????????????????????????С???顢????????????????????????????á??????????????????????????????????——Python ??? new ????? —— ???????? new ??????????????????????????????Щ?????????????繤???????????????????????
????# ????Vehicle ??????????????????????????!
????# ?????????????????以??????????????δ???
????car = Vehicle(wheels=4?? doors=4)
????????ü?????????????????????????????κ??????檔?????????????????????京???е???Python ?????? class ?? def ???????????????е??????С?Python ?????????????У?class ?? def ????????????????????????????????????????????????????
???????????????????????????????????????type??????????????????????????С??????????????????????????
????????ν??????????о??е??????????????£?????????????????????????е?????磬Python???enum?飬???????? enum module:
class Animal(Enum):
????cat = 0
????dog = 1
????mouse = 2
????snake = 3
????print(Animal.cat) # <Animal.cat: 0>
????print(Animal.cat.value) # 0
????print(Animal(2)) # <Animal.mouse: 2>
????print(Animal['dog']) # <Animal.dog: 1>
????class ??????????????????ζ???????????????????????????????д?ù???????????????????????Enum????????????????????????????????????????Щ??????????? Python ????????? Python ???????
??????????????????·???????????????????????е?????? self.foo = foo ???????????????????????????????????????????????б??Java ?????????????????????????? Amber ?????Python ?????????????????? attrs ?????????????
??import attr
????@attr.s
????class Point:
????x = attr.ib()
????y = attr.ib()
????p = Point(3?? 4)
????q = Point(x=3?? y=4)
????p == q # True?? which it wouldn't have been before!
????print(p) # Point(x=3?? y=4)
??????????? SQLAlchemy ??????????? Python ????????????????????????? Hibernate ?? ORM????????????????????????????????????????????????????????д???????????
????class Order(Table):
????id = Column(Integer?? primary_key=True)
????order_number = Column(Integer?? index=True)
????status = Column(Enum('pending'?? 'complete')?? default='pending')
????...
?????????????????Enum????SQLAlchemy???ú?property????????????????????????????λ???
????order.order_number = 5
????session.commit()
????????????????????б??????? ???е????????? thriftpy ?????????? module?? ?????????? Thrift ??????????? ??Java?У??????????????????????????μ?????裬??????2??????
??????????Щ?????????Python????????????????????????μ???塣????????????????Java?????????????в???????????????????????? — ??????Щ?????????д???????????????????bug??
????????
????Python ?к???Java?????????????????????????????????????Щ??μ????Java??????????????????Python??????????????????????????????????????????????????
????????????????Python????????Java???ó????????滻Java??Python???????????????????????(Pypy????????????Python)??Java??ж??????????????Python??????????Щ???????????????к?????????????????????????????????(????mypy?????Python???????????)??
????????Щ Java ?????ó???????????Python???????????????????????????????????????????У??????????????????????????????????? Python ????????????????????????е??????????????????????е?????????????????????ζ???????????????????????????????????????????????????????Щ?????????????????????? ??????? goto ?????
????????????????? Python ??????????????????????л?ú????????????????????????????????????????
????????????????и? Pyjnius ????????????????
from jnius import autoclass
????System = autoclass('java.lang.System')
????System.out.println('Hello?? world!')
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
Java???????????Щ???????????????Java????????????????Java?б???Map????????Java Web???????????????Java??????????????д?????Java????????7???????????????????????(java .net ?????)Java webdriver??λ????????′????е?????Java??д??????????????????Java???????????????JavaScript????????????Java?????????????????? Java???????10??????????????Java?м????????????????java???????ü???????????м???????????????????Java??α??????????
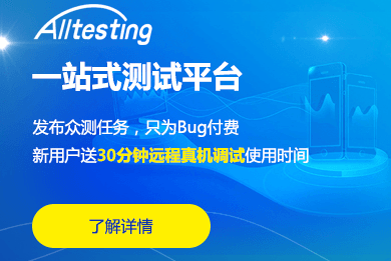
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????