java???????????????????
???????????? ???????[ 2017/3/15 10:16:59 ] ??????????????????? Java
????0??????
??????????????????????????????????????????????????????????????????????????????????????????????????????????
????1?????API????
????public final void notify()
??????????????????????????????????????????????????????????????????????????????
????public final void wait()
???????????????д?????????????????????????????????????????????????????????? notify ???????? notifyAll ?????????????????????????????????????????????????????????????????????????С?
????“????????????????????????????JVM??????????????????????????????????????????????????????”??
????wait()??notify()??notifyAll()????Щ?????????????????????У?????????м????????????в???????????????????У????????????????????????????????????????????????????????????????????????????????????????????????????????????????object???С?
????2 ?????????
?????????
????//???????????this??
????public class Storage<T> {
????// ????洢??
????private final int MAX_SIZE = 1000;
????private int storageSize = 100;
????private Object[] data;
????private int top = 0;
????public Storage(int size) {
????size = size <= 0 ? storageSize : size;
????size = size > this.MAX_SIZE ? this.MAX_SIZE : size;
????this.storageSize = size;
????data = new Object[this.storageSize];
????}
????public int getStorageSize() {
????return storageSize;
????}
????public synchronized T pop() {
????while (top == 0) {
????try {
????//???
????wait();
????} catch (Exception ex) {
????ex.printStackTrace();
????}
????}
????top--;
????T t = (T) data[top];
????//???????????
????notifyAll();
????return t;
????}
????public synchronized void push(T t) {
????while (top == storageSize) {
????try {
????wait();
????} catch (Exception ex) {
????ex.printStackTrace();
????}
????}
????data[top++] = t;
????notifyAll();
????}
????}
??????????
????public class Producer implements Runnable {
????private Storage<String> storage;
????private volatile boolean breakLoop = false;
????public Producer(Storage<String> storage) {
????this.storage = storage;
????}
????@Override
????public void run() {
????int index = 1;
????while (true) {
????if (breakLoop) {
????break;
????}
????try {
????Thread.sleep(1000);
????} catch (Exception ex) {
????ex.printStackTrace();
????}
????storage.push("data:" + index);
????System.out.println("Producer data:" + index);
????index++;
????}
????}
????public void destroy() {
????this.breakLoop = true;
????}
????}
??????????
????public class Consumer implements Runnable {
????private Storage<String> storage;
????private volatile boolean breakLoop = false;
????public Consumer(Storage<String> storage) {
????this.storage = storage;
????}
????@Override
????public void run() {
????while (true) {
????if (breakLoop) {
????break;
????}
????try {
????Thread.sleep(1000);
????} catch (Exception ex) {
????ex.printStackTrace();
????}
????System.out.println(Thread.currentThread().getName() + "Consumer:" + storage.pop());
????}
????}
????public void destroy() {
????this.breakLoop = true;
????}
????}
??????
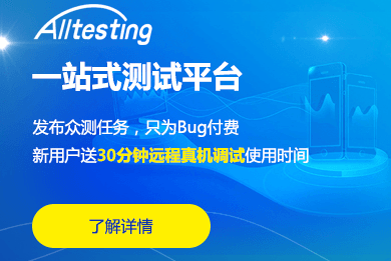
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11