???????????????
???????????? ???????[ 2016/12/1 10:45:04 ] ?????????????? .NET
????????????java???????????????????????????????????????????????????????
????????????????????????????????????????????????????????????????? ???????????????и??????????????????????????????????????
??????????????????????????
????* ???i=1??????i??????????????????[i/2];
????* ???2i > n??????i????????????????????2i;
????* ???2i+1 > n??????i????????????????????2i+1;
??????????????????????????????????????????????
????????????????????????????
????1. ???????????
????package org.sky.tree;
????/**
????* ??????????
????*
????* @author sky
????*
????* @param <E>
????*/
????public class BinaryTreeNode<E> {
????E element;
????BinaryTreeNode<E> leftChild;
????BinaryTreeNode<E> rightChild;
????public BinaryTreeNode() {
????super();
????this.element = null;
????this.leftChild = null;
????this.rightChild = null;
????}
????public BinaryTreeNode(E element) {
????super();
????this.element = element;
????this.leftChild = null;
????this.rightChild = null;
????}
????public BinaryTreeNode(E element?? BinaryTreeNode<E> leftChild??
????BinaryTreeNode<E> rightChild) {
????super();
????this.element = element;
????this.leftChild = leftChild;
????this.rightChild = rightChild;
????}
????public E getElement() {
????return element;
????}
????public void setElement(E element) {
????this.element = element;
????}
????public BinaryTreeNode<E> getLeftChild() {
????return leftChild;
????}
????public void setLeftChild(BinaryTreeNode<E> leftChild) {
????this.leftChild = leftChild;
????}
????public BinaryTreeNode<E> getRightChild() {
????return rightChild;
????}
????public void setRightChild(BinaryTreeNode<E> rightChild) {
????this.rightChild = rightChild;
????}
????public boolean isLeaf() {
????if (this.leftChild == null && this.rightChild == null) {
????return true;
????}
????return false;
????}
????}
????2. ???????????
????package org.sky.tree;
????import java.util.Collection;
????import java.util.LinkedList;
????import java.util.List;
????import java.util.Queue;
????import java.util.Scanner;
????import java.util.Stack;
????import java.util.concurrent.LinkedBlockingDeque;
????import java.util.concurrent.LinkedBlockingQueue;
????/**
????* ???????????
????*
????* @author sky
????*
????* @param <E>
????*/
????public class BinaryTree<E> {
????private BinaryTreeNode<E> root;
????public BinaryTree() {
????super();
????this.root = new BinaryTreeNode<E>();
????}
????public boolean isEmpty() {
????if (root == null) {
????return true;
????}
????return false;
????}
????public BinaryTreeNode<E> getRoot() {
????return root;
????}
????/**
????* ????????????????????????????????????????????
????* @param node
????* @param element
????*/
????public void createTreeRandomly(BinaryTreeNode<E> node?? E element){
????if(root == null){
????root = new BinaryTreeNode<E>();
????}else{
????if(Math.random() > 0.5){
????if(node.leftChild == null){
????node.leftChild = new BinaryTreeNode<E>(element);
????}else{
????createTreeRandomly(node.leftChild??element);
????}
????}else{
????if(node.rightChild == null){
????node.rightChild = new BinaryTreeNode<E>(element);
????}else{
????createTreeRandomly(node.rightChild??element);
????}
????}
????}
????}
????/**
????* ???????????????????????
????* ?????????????????????????????????????i=1??????i??????????????????[i/2];
????* ???2i > n??????i????????????????????2i;
????* ???2i+1 > n??????i????????????????????2i+1;
????* @param c
????*/
????private BinaryTreeNode<E> node = null;
????public void createCompleteBinaryTree(Collection<? extends E> c){
????if(c != null && c.size() > 0){
????List<BinaryTreeNode<E>> treeList = new LinkedList<BinaryTreeNode<E>>();
????for(Object o : c){
????BinaryTreeNode<E> binaryTreeNode = new BinaryTreeNode<E>((E)o);
????treeList.add(binaryTreeNode);
????}
????LinkedBlockingDeque<BinaryTreeNode<E>> queue = new LinkedBlockingDeque<BinaryTreeNode<E>>();
????//???treeList.size()/2 - 1????????????????????????????????????
????for(int parentIndex = 0; parentIndex < treeList.size()/2; parentIndex++){
????if(parentIndex == 0){
????root = treeList.get(parentIndex);
????//??????
????root.leftChild = treeList.get(parentIndex*2 + 1);
????queue.add(root.leftChild);
????//??????
????root.rightChild = treeList.get(parentIndex*2 +2);
????queue.add(root.rightChild);
????}else{
????if(!queue.isEmpty() && parentIndex*2+1 < treeList.size()){
????node = (BinaryTreeNode<E>) queue.poll();
????if(parentIndex*2+1 < treeList.size()){
????//??????
????node.leftChild = treeList.get(parentIndex*2 + 1);
????queue.add(root.leftChild);
????}
????if(parentIndex*2+2 < treeList.size()){
????//??????
????node.rightChild = treeList.get(parentIndex*2 + 2);
????queue.add(root.rightChild);
????}
????}else{
????return ;
????};
????}
????}
????}
????}
????/**
????* ????????????????????????????‘#’???????;
????* ????????input.txt:- + a # # * # # / e # # f # #;
????* @param inputFile
????*/
????public void preOrderCreateBinaryTree(String inputFile){
????Scanner scanner = null;
????try{
????scanner = new Scanner(inputFile);
????}catch(Exception e){
????e.printStackTrace();
????}
????this.root = preOrderCreateBinaryTree(root?? scanner);
????}
????public BinaryTreeNode<E> preOrderCreateBinaryTree(BinaryTreeNode<E> node?? Scanner scanner){
????String temp = scanner.next();
????if(temp.trim().equals("#")){
????return null;
????}else{
????node = new BinaryTreeNode<E>((E)temp);
????node.setLeftChild(preOrderCreateBinaryTree(node.getLeftChild()?? scanner));
????node.setRightChild(preOrderCreateBinaryTree(node.getRightChild()?? scanner));
????return node;
????}
????}
????/**
????* ??????????????????????????????????
????* ??飺???????????????????????????????????????????????????????????????????
????*/
????/**
????* ????????????????
????* @param root
????* @return ????????????
????*/
????public int getNodeNumber(BinaryTreeNode<E> root){
????if(root == null){
????return 0;
????}else{
????return getNodeNumber(root.leftChild) + getNodeNumber(root.rightChild) + 1;
????}
????}
????/**
????* ???????????????
????* @param root
????* @return ???????????
????*/
????public int getDepth(BinaryTreeNode<E> root){
????if(root == null){
????return 0;
????}else{
????int depthLeft = getDepth(root.leftChild); //?????????
????int depthRight = getDepth(root.rightChild); //?????????
????return depthLeft > depthRight ? (depthLeft+1) : (depthRight+1);
????}
????}
????/**
????* ????????????k????????
????* @param root
????* @param k
????* @return ??k????????
????*/
????public int getKthLevelNodeNumber(BinaryTreeNode<E> root?? int k){
????if(root == null || k < 1){
????return 0;
????}
????if(k == 1){
????return 1;
????}
????int leftNumber = getKthLevelNodeNumber(root.leftChild?? k-1);
????int rightNumber = getKthLevelNodeNumber(root.rightChild??k-1);
????return (leftNumber + rightNumber);
????}
????/**
????* ???????????????????
????* @param root
????* @return
????*/
????public int getLeafNodeNumber(BinaryTreeNode<E> root){
????if(root == null){
????return 0;
????}
????if(root.leftChild == null && root.rightChild == null){
????return 1;
????}
????int leftNumber = getLeafNodeNumber(root.leftChild);
????int rightNumber = getLeafNodeNumber(root.rightChild);
????return (leftNumber + rightNumber);
????}
????/**
????* ?ж???????????????????
????* ??????
????* ??1??????????????????????????
????* ??2?????????????????????????ò??????????
????* ??3??????????????????????????????????????????????????????棬?????????
????* @param root1
????* @param root2
????* @return ????????????true;
????*/
????public boolean strutureComp(BinaryTreeNode<E> root1?? BinaryTreeNode<E> root2){
????if(root1 == null && root2 == null){
????return true;
????}else if(root1 == null || root2 == null){
????return false;
????}
????boolean leftResult = strutureComp(root1.leftChild?? root2.leftChild);
????boolean rightResult = strutureComp(root1.rightChild?? root2.rightChild);
????return (leftResult && rightResult);
????}
????/**
????* ????????
????* @param root
????* @param element
????* @return ??????
????*/
????public BinaryTreeNode<E> insert(BinaryTreeNode<E> root?? E element){
????BinaryTreeNode<E> pointer = new BinaryTreeNode<E>(element);
????if(root == null){
????root = pointer;
????}else if(root.leftChild == null){
????root.leftChild = insert(root.leftChild?? element);
????}else if(root.rightChild == null){
????root.rightChild = insert(root.rightChild?? element);
????}
????return root;
????}
??????
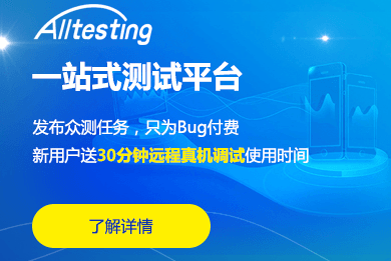
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11