???XML??????????????????
???????????? ???????[ 2015/1/5 11:08:33 ] ???????????XML ????? ???????
public T SelectById(string id)
{
Type t = typeof(T);
foreach (var inst in this.entityList)
{
foreach (PropertyInfo pro in t.GetProperties())
{
if (pro.Name.ToLower() == "id")
{
if (id == (pro.GetValue(inst?? null) ?? string.Empty).ToString())
{
return inst;
}
}
}
}
return default(T);
}
public void UpdateById(T entity)
{
Type t = typeof(T);
string id = string.Empty;
foreach (PropertyInfo pro in t.GetProperties())
{
if (pro.Name.ToLower() == "id")
{
id = (pro.GetValue(entity?? null) ?? string.Empty).ToString();
break;
}
}
this.DeleteById(id);
this.Insert(entity);
}
public void DeleteById(string id)
{
Type t = typeof(T);
T entity = default(T);
foreach (var inst in this.entityList)
{
foreach (PropertyInfo pro in t.GetProperties())
{
if (pro.Name.ToLower() == "id")
{
if ((pro.GetValue(inst?? null) ?? string.Empty).ToString() == id)
{
entity = inst;
goto FinishLoop;
}
}
}
}
FinishLoop:
this.entityList.Remove(entity);
this.WriteDb();
}
public List<T> SelectAll()
{
this.ReadDb();
return this.entityList;
}
public void DeleteAll()
{
this.entityList.Clear();
this.WriteDb();
}
private void WriteDb()
{
XmlSerializer ks = new XmlSerializer(typeof(List<T>));
FileInfo fi = new FileInfo(this.Dbfile);
var dir = fi.Directory;
if (!dir.Exists)
{
dir.Create();
}
Stream writer = new FileStream(this.Dbfile?? FileMode.Create?? FileAccess.ReadWrite);
ks.Serialize(writer?? this.entityList);
writer.Close();
}
private void ReadDb()
{
if (File.Exists(this.Dbfile))
{
XmlSerializer ks = new XmlSerializer(typeof(List<T>));
Stream reader = new FileStream(this.Dbfile?? FileMode.Open?? FileAccess.ReadWrite);
this.entityList = ks.Deserialize(reader) as List<T>;
reader.Close();
}
else
{
this.entityList = new List<T>();
}
}
}
}
using System.Collections.Generic;
namespace Wisdombud.xmldb
{
public class BaseXmlBll<T> where T : new()
{
public string DbFile
{
get { return this.bll.Dbfile; }
set { bll.Dbfile = value; }
}
private XmlSerializerBll<T> bll = XmlSerializerBll<T>.GetInstance();
public void Delete(string id)
{
var entity = this.Select(id);
bll.DeleteById(id);
}
public void Insert(T entity)
{
bll.Insert(entity);
}
public void Insert(List<T> list)
{
bll.InsertRange(list);
}
public System.Collections.Generic.List<T> SelectAll()
{
return bll.SelectAll();
}
public void Update(string oldId?? T entity)
{
bll.UpdateById(entity);
}
public T Select(string id)
{
return bll.SelectById(id);
}
public System.Collections.Generic.List<T> SelectBy(string name?? object value)
{
return bll.SelectBy(name?? value);
}
public void DeleteAll()
{
bll.DeleteAll();
}
}
}
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
?????????????????????????Щ????????????????????TC???????????????Щ???????????????????????????????????????????????(java .net ?????)???mysql???????????????????ж????д???Python???????????????(DB2)??????BufferPool????????????????????????????????6??????????????????滮???????????????-????????SQL Server???????????????????λ?????PHP??SQL????????????????????Pythonд???NoSQL????????? SQL ?е????????????? SQL ?е?????????Java???????:?????MySQL???????
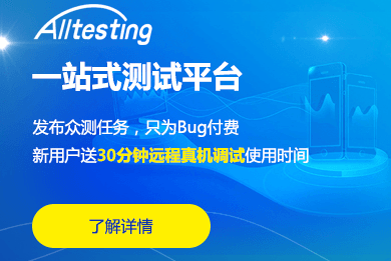
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????