C#??ζ???Excel???沢????????????(??)
???????????? ???????[ 2015/12/14 11:49:20 ] ????????????? Excel
???????????????:
????1 using System;
????2 using System.Collections.Generic;
????3 using System.Linq;
????4 using System.Text;
????5 using Microsoft.Office.Tools.Ribbon;
????6
????7 namespace ExcelAddInDemo
????8 {
????9 public partial class RibbonAddIn
????10 {
????11
????12 private void RibbonAddIn_Load(object sender?? RibbonUIEventArgs e)
????13 {
????14
????15 }
????16
????17 private void btnAbout_Click(object sender?? RibbonControlEventArgs e)
????18 {
????19 System.Windows.Forms.MessageBox.Show("JackWangCUMT!");
????20 }
????21
????22 private void btnShow_Click(object sender?? RibbonControlEventArgs e)
????23 {
????24 if (Globals.ThisAddIn._MyCustomTaskPane != null)
????25 {
????26 Globals.ThisAddIn._MyCustomTaskPane.Visible = true;
????27 }
????28 }
????29
????30 private void btnHide_Click(object sender?? RibbonControlEventArgs e)
????31 {
????32 if (Globals.ThisAddIn._MyCustomTaskPane != null)
????33 {
????34 Globals.ThisAddIn._MyCustomTaskPane.Visible = false;
????35 }
????36 }
????37 }
????38 }
????3 ThisAddIn?????д
????1 using System;
????2 using System.Collections.Generic;
????3 using System.Linq;
????4 using System.Text;
????5 using System.Xml.Linq;
????6 using Excel = Microsoft.Office.Interop.Excel;
????7 namespace ExcelAddInDemo
????8 {
????9 using Microsoft.Office.Tools;
????10 public partial class ThisAddIn
????11 {
????12 public CustomTaskPane _MyCustomTaskPane = null;
????13
????14 private void ThisAddIn_Startup(object sender?? System.EventArgs e)
????15 {
????16 UCTaskPane taskPane = new UCTaskPane();
????17 _MyCustomTaskPane = this.CustomTaskPanes.Add(taskPane?? "??????????");
????18 _MyCustomTaskPane.Width = 30;//height?????????width ==height
????19 _MyCustomTaskPane.Visible = true;
????20 _MyCustomTaskPane.DockPosition = Microsoft.Office.Core.MsoCTPDockPosition.msoCTPDockPositionTop;
????21
????22 UCPaneLeft panLeft = new UCPaneLeft();
????23 _MyCustomTaskPane = this.CustomTaskPanes.Add(panLeft?? "???");
????24 _MyCustomTaskPane.Width = 200;
????25 _MyCustomTaskPane.Visible = true;
????26 _MyCustomTaskPane.DockPosition = Microsoft.Office.Core.MsoCTPDockPosition.msoCTPDockPositionLeft;
????27
????28 UCTaskGrid panRight = new UCTaskGrid();
????29 _MyCustomTaskPane = this.CustomTaskPanes.Add(panRight?? "????б?");
????30 _MyCustomTaskPane.Width = 200;
????31 _MyCustomTaskPane.Visible = true;
????32 _MyCustomTaskPane.DockPosition = Microsoft.Office.Core.MsoCTPDockPosition.msoCTPDockPositionRight;
????33
????34 UCLog panLog = new UCLog();
????35 _MyCustomTaskPane = this.CustomTaskPanes.Add(panLog?? "????б?");
????36 _MyCustomTaskPane.Width = 60;
????37 _MyCustomTaskPane.Visible = true;
????38 _MyCustomTaskPane.DockPosition = Microsoft.Office.Core.MsoCTPDockPosition.msoCTPDockPositionBottom;
????39
????40 //Hook into the workbook open event
????41 //This is because Office doesn't always have a document ready when this method is run
????42 this.Application.WorkbookActivate += Application_WorkbookActivate;
????43 //test
????44 //this.Application.SheetSelectionChange += Application_SheetSelectionChange;
????45 }
????46
????47 void Application_SheetSelectionChange(object Sh?? Excel.Range Target)
????48 {
????49 if (this.Application != null)
????50 {
????51 this.Application.Caption = this.Application.ActiveCell.Address.ToString();//$A$1
????52 //+ this.Application.ActiveCell.AddressLocal.ToString();//$A$1
????53 //this.Application.ActiveCell.Formula = "=sum(1+2)";
????54
????55 }
????56 }
????57
????58 void Application_WorkbookActivate(Excel.Workbook Wb)
????59 {
????60 //using Microsoft.Office.Tools.Excel ?? using Microsoft.Office.Interop.Excel ????worksheet??????????
????61 //string path = this.Application.ActiveWorkbook.FullName;
????62 Excel._Worksheet ws = (Excel._Worksheet)this.Application.ActiveWorkbook.ActiveSheet;
????63 ws.Cells[2?? 2] = "ID2";
????64 //?????????????д??о?
????65 int r=2??c=2;
????66 //((Excel.Range)ws.Cells[r?? c]).NumberFormat = format;
????67 ((Excel.Range)ws.Cells[r?? c]).Value2 = "ID";
????68 ((Excel.Range)ws.Cells[r?? c]).Interior.Color =System.Drawing. ColorTranslator.ToOle(System.Drawing.Color.Red);
????69 //((Excel.Range)ws.Cells[r?? c]).Style.Name = "Normal";
????70 ((Excel.Range)ws.Cells[r?? c]).Style.Font.Bold = true;
????71
????72 #region format
????73 ((Microsoft.Office.Interop.Excel.Range)ws.get_Range("A2"?? "E10")).Font.Bold = true;
????74 ((Microsoft.Office.Interop.Excel.Range)ws.get_Range("A2"?? "E10")).Font.Italic = true;
????75 ((Microsoft.Office.Interop.Excel.Range)ws.get_Range("A2"?? "E10")).Font.Color = System.Drawing.Color.FromArgb(96?? 32?? 0).ToArgb();
????76 ((Microsoft.Office.Interop.Excel.Range)ws.get_Range("A2"?? "E10")).Font.Name = "Calibri";
????77 ((Microsoft.Office.Interop.Excel.Range)ws.get_Range("A2"?? "E10")).Font.Size = 15;
????78
????79 //border
????80 Excel.Range range = ((Microsoft.Office.Interop.Excel.Range)ws.get_Range("B2"?? "E3"));
????81 Excel. Borders border = range.Borders;
????82 border[Excel.XlBordersIndex.xlEdgeBottom].LineStyle =Excel. XlLineStyle.xlContinuous;
????83 border.Weight = 2d;
????84 border[Excel.XlBordersIndex.xlEdgeTop].LineStyle = Excel.XlLineStyle.xlContinuous;
????85 border[Excel.XlBordersIndex.xlEdgeLeft].LineStyle = Excel.XlLineStyle.xlContinuous;
????86 border[Excel.XlBordersIndex.xlEdgeRight].LineStyle = Excel.XlLineStyle.xlContinuous;
????87 #endregion
????88 ws.Cells[2?? 4] = "First";
????89 ws.Cells[3?? 2] = "Last";
????90 ws.Cells[3?? 4] = "Email";
????91 }
????92 private void ThisAddIn_Shutdown(object sender?? System.EventArgs e)
????93 {
????94 }
????95
????96 #region VSTO ????????
????97
????98 /// <summary>
????99 /// ???????????????? - ???
????100 /// ???????????????????????
????101 /// </summary>
????102 private void InternalStartup()
????103 {
????104 this.Startup += new System.EventHandler(ThisAddIn_Startup);
????105 this.Shutdown += new System.EventHandler(ThisAddIn_Shutdown);
????106 }
????107
????108 #endregion
????109 }
????110 }
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
?????????????????????????Щ????????????????????TC???????????????Щ???????????????????????????????????????????????(java .net ?????)???mysql???????????????????ж????д???Python???????????????(DB2)??????BufferPool????????????????????????????????6??????????????????滮???????????????-????????SQL Server???????????????????λ?????PHP??SQL????????????????????Pythonд???NoSQL????????? SQL ?е????????????? SQL ?е?????????Java???????:?????MySQL???????
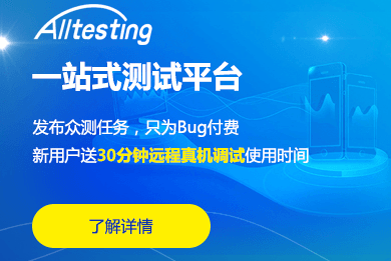
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????