VC??ADO??????????????
???????????? ???????[ 2014/8/4 11:43:41 ] ????????????? ????
/**//**************************************************************
CPFile.cpp
**************************************************************/
/**///////////////////////////////////////////////////////////////////////
// ??????
/**///////////////////////////////////////////////////////////////////////
//?????????
CPData::CPData()
{
//?????
m_nResultRow = 0;
m_nResultCol=0;
m_pConnection = NULL;
//????????
m_pRecordset.CreateInstance(_uuidof(Recordset));
m_pCommand.CreateInstance(_uuidof(Command));
}
//??????????????
CPData::CPData(_ConnectionPtr pConnection)
{
m_pConnection = pConnection;
m_nResultRow = 0;
m_nResultCol=0;
//????????
m_pRecordset.CreateInstance(_uuidof(Recordset));
m_pCommand.CreateInstance(_uuidof(Command));
}
/**///////////////////////////////////////////////////////////////////////
// ????????
/**///////////////////////////////////////////////////////////////////////
CPData::~CPData()
{
if(m_pRecordset->State!=adStateClosed)
m_pRecordset->Close();
m_pRecordset = NULL;
if(m_pCommand->State!=adStateClosed)
m_pCommand->Release();
m_pCommand = NULL;
if(m_pConnection->State!=adStateClosed)
m_pConnection->Close();
m_pConnection = NULL;
}
/**//////////////////////////////////////////////////////////////////////
///??????????
////////////////////////////////////////////////////////////////////
//????????????????
long CPData::GetResultRow()
{
return this->m_nResultRow;
}
//????????????????
long CPData::GetResultCol()
{
return this->m_nResultCol;
}
//??????????
_RecordsetPtr CPData::GetResult()
{
return this->m_pRecordset;
}
/**////////////////////////////////////////////////////////////////////////
///???????
///////////////////////////////////////////////////////////////////////
//??????????
//1.??????????????????????????????ж???
BOOL CPData::Connect(CString strUser??CString strPassword??CString strFileName??int nOptions??CString strConStr)
{
try{
m_pConnection.CreateInstance(__uuidof(Connection));
HRESULT hr;
//??????????????????????????????
if(strFileName.Compare("")!=0&&CPFile::IsFileExist(strFileName))
{
CString con = "File Name="+strFileName;
m_pConnection->ConnectionString =(_bstr_t)con;
hr=m_pConnection->Open(""??""??""??nOptions);
}
else
{
//????????????????
m_pConnection->ConnectionString = (_bstr_t)strConStr;
hr=m_pConnection->Open(""??_bstr_t(strUser)??_bstr_t(strPassword)??nOptions);
}
//????????
//???????
if(FAILED(hr))
{
AfxMessageBox("???????!");
return FALSE;
}
}
catch(_com_error&e)
{
AfxMessageBox(e.Description()+"B");
return FALSE;
}
return TRUE;
}
//???????
void CPData::DisConnect()
{
if(m_pConnection->State!=adStateClosed)
m_pConnection->Close();
}
/**////////////////////////////////////////////////////////////////////////
///???2???
///////////////////////////////////////////////////////////////////////
BOOL CPData::Execute(CString strSql)
{
try
{
_variant_t vNULL;
vNULL.vt = VT_ERROR;
/**////??????????
vNULL.scode = DISP_E_PARAMNOTFOUND;
/**////?????????????????????????????
m_pCommand->ActiveConnection = m_pConnection;
/**////???????
m_pCommand->CommandText = (_bstr_t)strSql;
/**////?????????ü????
m_pRecordset = m_pCommand->Execute(&vNULL??&vNULL??adCmdText);
//????vNULL?е?intVal????в?????????????
m_nResultRow = 0;
m_nResultRow = vNULL.intVal;
}
catch(_com_error&e)
{
m_nResultRow = 0;
return FALSE;
}
return TRUE;
}
/**////////////////////////////////////////////////////////////////////////
///???????
///////////////////////////////////////////////////////////////////////
BOOL CPData::Select(CString strSql)
{
try
{
m_nResultCol=0;
m_nResultRow=0;
m_pRecordset->CursorLocation=adUseClient; //?????α?λ??????????????????????GetRecordCount()?????????
m_pRecordset->Open(_variant_t(strSql)??_variant_t((IDispatch *)m_pConnection??true)??adOpenDynamic??adLockOptimistic??adCmdText);
m_nResultCol = m_pRecordset->Fields->GetCount();//???????????????
m_nResultRow = m_pRecordset->GetRecordCount(); //???????????????
}
catch(_com_error&e)
{
AfxMessageBox(e.Description()+"D");
return FALSE;
}
return TRUE;
}
//??????????????????????ε???????д???
//????????CStringArray????????pResult??
BOOL CPData::Select(CString strSql??CStringArray& Result)
{
if(Select(strSql)!=0)
{
Result.RemoveAll();
for(int i=0;i<m_nResultRow;i++)
{
_variant_t value;
value=m_pRecordset->Fields->Item[(long)0]->Value;
if(value.vt==3||value.vt==14)
{
CString strTrans;
strTrans.Format("%ld"??value.intVal);
Result.Add(strTrans);
}
else
Result.Add(value.bstrVal);//
m_pRecordset->MoveNext();
}
m_pRecordset->Close();
return TRUE;
}
else
{
m_pRecordset->Close();
return FALSE;
}
}
BOOL CPData::SelectMulitCol(CString strSql??CStringArray& Result)
{
if(Select(strSql)!=0)
{
Result.RemoveAll();
_variant_t value;
for(int i=0;i<m_nResultRow;i++)
{
for(int j=0;j<m_nResultCol;j++)
{
value=m_pRecordset->Fields->Item[(long)(/**//*i*m_nResultCol+*/j)]->Value;
if(value.vt==3||value.vt==14)
{
CString strTrans;
strTrans.Format("%ld"??value.intVal);
Result.Add(strTrans);
}
else
if(value.vt==7)
{
COleDateTime time = value.date;
CString strTemp;
strTemp.Format("%d-%d-%d %s"??time.GetYear()??time.GetMonth()??time.GetDay()??time.Format("%H:%M:%S"));
Result.Add(strTemp);
}
else
Result.Add(value.bstrVal);//
}
m_pRecordset->MoveNext();
}
m_pRecordset->Close();
return TRUE;
}
else
{
m_pRecordset->Close();
return FALSE;
}
}
//???????
BOOL CPData::OpenTable(CString strTable)
{
try
{
m_nResultCol=0;
m_nResultRow=0;
m_pRecordset->CursorLocation=adUseClient; //?????α?λ??????????????????????GetRecordCount()?????????
m_pRecordset->Open(_variant_t(strTable)??_variant_t((IDispatch *)m_pConnection??true)??adOpenDynamic??adLockOptimistic??adCmdTable);
m_nResultCol = m_pRecordset->Fields->GetCount();//???????????????
m_nResultRow = m_pRecordset->GetRecordCount(); //???????????????
}
catch(_com_error&e)
{
AfxMessageBox(e.Description()+"E");
return FALSE;
}
return TRUE;
}
BOOL CPData::OpenTable(CString strTable??CStringArray& Result)
{
if(OpenTable(strTable)!=0)
{
Result.RemoveAll();
_variant_t value;
for(int i=0;i<m_nResultRow;i++)
{
for(int j=0;j<m_nResultCol;j++)
{
value=m_pRecordset->Fields->Item[(long)(/**//*i*m_nResultCol+*/j)]->Value;
if(value.vt==3||value.vt==14)
{
CString strTrans;
strTrans.Format("%ld"??value.intVal);
Result.Add(strTrans);
}
else
if(value.vt==7)
{
COleDateTime time = value.date;
CString strTemp;
strTemp.Format("%d-%d-%d %s"??time.GetYear()??time.GetMonth()??time.GetDay()??time.Format("%H:%M:%S"));
Result.Add(strTemp);
}
else
Result.Add(value.bstrVal);//
}
m_pRecordset->MoveNext();
}
m_pRecordset->Close();
return TRUE;
}
else
{
return FALSE;
}
}
/**//////////////////////////////////////////////////////////////////////////////
///???????
/////////////////////////////////////////////////////////////////////////////
void CPData::CloseRecordset()
{
if(m_pRecordset->State != adStateClosed)
m_pRecordset->Close();
}
BOOL CPData::ExecuteTrans(CStringArray& aSql)
{
try{
int nNum = aSql.GetSize();
m_pConnection->BeginTrans();
for(int i=0;i<nNum;i++)
{
CString strSql = aSql.GetAt(i);
m_pConnection->Execute((_bstr_t)aSql.GetAt(i)??NULL??adCmdText);
}
m_pConnection->CommitTrans();
return TRUE;
}
catch(_com_error& e)
{
m_pConnection->RollbackTrans();
AfxMessageBox(e.Description()+"F");
return FALSE;
}
}
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
?????????????????????????Щ????????????????????TC???????????????Щ???????????????????????????????????????????????(java .net ?????)???mysql???????????????????ж????д???Python???????????????(DB2)??????BufferPool????????????????????????????????6??????????????????滮???????????????-????????SQL Server???????????????????λ?????PHP??SQL????????????????????Pythonд???NoSQL????????? SQL ?е????????????? SQL ?е?????????Java???????:?????MySQL???????
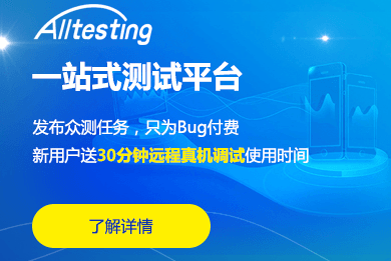
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????