???????????
???????????? ???????[ 2014/7/1 11:22:45 ] ?????????????
public static Connection getConnection() throws Exception
{
InitialContext cxt = new InitialContext();
if(cxt == null)
{
throw new Exception("no context!");
}
DataSource ds = (DataSource) cxt.lookup("java:/comp/env/jdbc/orcl");
if(ds == null)
{
throw new Exception("Data source not found!");
}
return ds.getConnection();
}
public static PreparedStatement getPreparedStatement(Connection conn?? String sql) throws Exception
{
if(conn == null || sql == null || sql.trim().equals(""))
{
return null;
}
PreparedStatement pstmt = conn.prepareStatement(sql.trim());
return pstmt;
}
public static void setPreparedStatementParam(PreparedStatement pstmt?? List<Object> paramList) throws Exception
{
if(pstmt == null || paramList == null || paramList.isEmpty())
{
return;
}
DateFormat df = DateFormat.getDateTimeInstance();
for(int i = 0; i < paramList.size(); i++)
{
if(paramList.get(i) instanceof Integer)
{
int paramValue = ((Integer) paramList.get(i)).intValue();
pstmt.setInt(i + 1?? paramValue);
}
else if(paramList.get(i) instanceof Float)
{
float paramValue = ((Float) paramList.get(i)).floatValue();
pstmt.setFloat(i + 1?? paramValue);
}
else if(paramList.get(i) instanceof Double)
{
double paramValue = ((Double) paramList.get(i)).doubleValue();
pstmt.setDouble(i + 1?? paramValue);
}
else if(paramList.get(i) instanceof Date)
{
pstmt.setString(i + 1?? df.format((Date)paramList.get(i)));
}
else if(paramList.get(i) instanceof Long)
{
long paramValue = ((Long)paramList.get(i)).longValue();
pstmt.setLong(i + 1?? paramValue);
}
else if(paramList.get(i) instanceof String)
{
pstmt.setString(i + 1?? (String)paramList.get(i));
}
}
return;
}
private static void closeConn(Connection conn)
{
if(conn == null)
{
return;
}
try
{
conn.close();
}
catch(SQLException e)
{
logger.info(e.getMessage());
}
}
private static void closeStatement(Statement stmt)
{
if(stmt == null)
{
return;
}
try
{
stmt.close();
}
catch(SQLException e)
{
logger.info(e.getMessage());
}
}
private static void closeResultSet(ResultSet rs)
{
if(rs == null)
{
return;
}
try
{
rs.close();
}
catch(SQLException e)
{
logger.info(e.getMessage());
}
}
private static ResultSet getResultSet(PreparedStatement pstmt) throws Exception
{
if(pstmt == null)
{
return null;
}
ResultSet rs = pstmt.executeQuery();
return rs;
}
public static List<Map<String??String>> getQueryList(String sql?? List<Object> paramList) throws Exception
{
if(sql == null || sql.trim().equals(""))
{
logger.info("parameter is valid!");
return null;
}
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
List<Map<String??String>> queryList = null;
try
{
conn = DbUtils.getConnection();
pstmt = DbUtils.getPreparedStatement(conn?? sql);
setPreparedStatementParam(pstmt?? paramList);
if(pstmt == null)
{
return null;
}
rs = DbUtils.getResultSet(pstmt);
queryList = DbUtils.getQueryList(rs);
}
catch(Exception e)
{
logger.info(e.getMessage());
throw new Exception();
}
finally
{
closeResultSet(rs);
closeStatement(pstmt);
closeConn(conn);
}
return queryList;
}
private static List<Map<String??String>> getQueryList(ResultSet rs) throws Exception
{
if(rs == null)
{
return null;
}
ResultSetMetaData rsMetaData = rs.getMetaData();
int columnCount = rsMetaData.getColumnCount();
List<Map<String??String>> dataList = new ArrayList<Map<String??String>>();
while(rs.next())
{
Map<String??String> dataMap = new HashMap<String??String>();
for(int i = 0; i < columnCount; i++)
{
dataMap.put(rsMetaData.getColumnName(i+1)?? rs.getString(i+1));
}
dataList.add(dataMap);
}
return dataList;
}
}
|
????????????????????????????????????ù??????????????????????????????????鷳???????????????????????????????????????????????鷳???????Hibernate????(????????????????)??
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
?????????????????????????Щ????????????????????TC???????????????Щ???????????????????????????????????????????????(java .net ?????)???mysql???????????????????ж????д???Python???????????????(DB2)??????BufferPool????????????????????????????????6??????????????????滮???????????????-????????SQL Server???????????????????λ?????PHP??SQL????????????????????Pythonд???NoSQL????????? SQL ?е????????????? SQL ?е?????????Java???????:?????MySQL???????
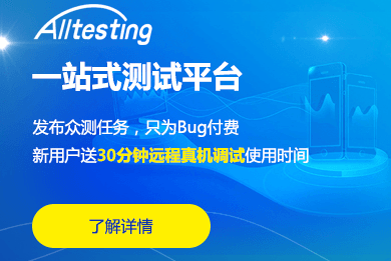
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????