Mybatis??ò???????????????
???????????? ???????[ 2014/6/18 10:56:48 ] ????????Mybatis ?????
//=================================???′????myBatis??????÷???(?????BySql)===========================//
/**
* ??????в?????
* @param statementId ????myBatis??mapper???????????????
* ????????mapper??namespace+"." + ??mapper??????ε?id
* @param value ??????????
* @return ????????????
*/
public int insertBySql(String statementId?? Object value) {
return sqlSession.insert(statementId?? value);
}
/**
* ???????е????????????????????????????????????
*
* @param statementId ????myBatis??mapper???????????????
* ????????mapper??namespace+"." + ??mapper??????ε?id
* @param value ????????
* @return ????????????
*/
public int deleteBySql(String statementId?? Object value) {
return sqlSession.delete(statementId?? value);
}
/**
* ???????е??????????????£?????????3??????????
*
* @param statementId ????myBatis??mapper???????????????
* ????????mapper??namespace+"." + ??mapper??????ε?id
* @param value ??????????
* @return ???3????????
*/
public int updateBySql(String statementId?? Object value) {
return sqlSession.update(statementId?? value);
}
/**
* ?????????????????????List?????
*
* @param statementId ????myBatis??mapper???????????????
* ????????mapper??namespace+"." + ??mapper??????ε?id
* @param value ????????
* @return list ???????
*/
public List selectListBySql(String statementId?? Object value) {
List list = sqlSession.selectList(statementId?? value);
//??????п???????(char????)??????myBatis???????????????У??????????????????????????
//???????????????????·??
//list = Config.getPropertyBool("po.propertyvalue.trimable"?? true) ? BeanUtil.trim(list) : list
return list;
}
/**
* ?????????????????????????Object????</br>
* @param statementId ????myBatis??mapper???????????????
* ????????mapper??namespace+"." + ??mapper??????ε?id
* @param parameter ????????
* @return list ???????
*/
public Object selectOneBySql(String statementId?? Object parameter) {
Object bean = sqlSession.selectOne(statementId?? parameter);
//??????п???????(char????)??????myBatis???????????????У??????????????????????????
//???????????????????·??
//bean = Config.getPropertyBool("po.propertyvalue.trimable"?? true) ? BeanUtil.trim(bean) : bean
return bean;
}
/**
* myBatis??????????????????
* <p>
* ???????÷???????????myBatis??mapper????д???statementId_COUNT???</br>
* <b>??????????????????????????????????????????????????????ResultSet??absolute??λ??????<br>
* ????????????????ResultSet???λ??????????????1???????????)??Ч??????<br>
* ???????Hibernate??query????????mapper??XML????????????????????<br>
* ??????pageId??pageSize???????????SQL????????limit n??m?У????????
* </b>
* </p>
* @param statementId ????myBatis??mapper???????????????????????mapper??namespace+"." + ??sqlMap??????ε?id
* @param parameter ???????????
* @param offset ???????????????У???0???
* @param pageSize ????????????????
* @throws com.fbd.crm.exception.BaseUncheckedException
* @return com.fbd.crm.common.QueryResult
*/
@SuppressWarnings({ "rawtypes"?? "unchecked" })
public QueryResult selectListForPagingBySql(String statementId?? Object parameter) throws Exception{
Assert.hasText(statementId?? "?????SQL????ID???????.");
//?????????
Integer count = null;
try {
count = (Integer)sqlSession.selectOne(statementId + "_COUNT"?? parameter);
} catch (Exception e) {
String msg = "????????в?????COUNT???????????????????????????. SqlMap id:" + statementId;
log.error(msg?? e);
throw new Exception(e);
}
if ((count == null) || (count.intValue() <= 0)) {
log.info("???COUNT????????????0???????SQL??????????????.?????????????????????????????.");
return new QueryResult(new ArrayList()?? 0);
}
List resultList = sqlSession.selectList(statementId?? parameter);
QueryResult result = new QueryResult(resultList?? count);
return result;
}
/**
* myBatis????????
* </b>
* </p>
* @param statementId ????myBatis??mapper???????????????????????mapper??namespace+"." + ??sqlMap??????ε?id
* @param parameter ???????????
* @throws com.fbd.crm.exception.BaseUncheckedException
* @return com.fbd.crm.common.QueryResult
*/
public QueryResult selectListWithTotal(String statementId?? Object parameter) throws Exception{
Assert.hasText(statementId?? "?????SQL????ID???????.");
//?????????
Integer count = null;
try {
count = (Integer)sqlSession.selectOne(statementId + "_COUNT"?? parameter);
} catch (Exception e) {
String msg = "????????в?????COUNT???????????????????????????. SqlMap id:" + statementId;
log.error(msg?? e);
throw new Exception(e);
}
if ((count == null) || (count.intValue() <= 0)) {
log.info("???COUNT????????????0???????SQL??????????????.?????????????????????????????.");
return new QueryResult(new ArrayList()??new ArrayList()?? 0);
}
List resultList = sqlSession.selectList(statementId?? parameter);
List totalList = sqlSession.selectList(statementId + "_TOTAL"?? parameter);
QueryResult result = new QueryResult(resultList?? totalList?? count);
return result;
}
/**
* ???sql
* @category ????????spring????????????
* @param sql
* @return List
* @throws SQLException
*/
public List executeQueryBySql(String sql) throws SQLException{
Connection con = null;
Statement stmt = null;
List list = new ArrayList();
try {
con = this.getConnection();//sqlSession.getConnection();
con.setAutoCommit(false);
stmt = con.createStatement(ResultSet.TYPE_SCROLL_INSENSITIVE??ResultSet.CONCUR_UPDATABLE);
ResultSet rSet = stmt.executeQuery(sql);
ResultSetMetaData md = rSet.getMetaData();
int num = md.getColumnCount();
while (rSet.next()) {
Map mapOfColValues = new HashMap(num);
for (int i = 1; i <= num; i++) {
mapOfColValues.put(md.getColumnName(i)?? rSet.getObject(i));
}
list.add(mapOfColValues);
}
con.commit();
} catch (Exception e) {
e.printStackTrace();
throw new SQLException("sql??????");
}finally {
if (stmt != null) {
stmt.close();
}
// ?????????????????org.springframework.jdbc.datasource.DataSourceTransactionManager??????
if (con != null) {
con.close();
}
}
return list;
}
}
|
???????????????????????漰???????????????????SPASVOС??(021-61079698-8054)?????????????????????????
??????
?????????????????????????Щ????????????????????TC???????????????Щ???????????????????????????????????????????????(java .net ?????)???mysql???????????????????ж????д???Python???????????????(DB2)??????BufferPool????????????????????????????????6??????????????????滮???????????????-????????SQL Server???????????????????λ?????PHP??SQL????????????????????Pythonд???NoSQL????????? SQL ?е????????????? SQL ?е?????????Java???????:?????MySQL???????
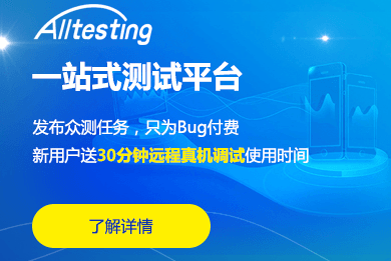
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11????????
?????????App Bug???????????????????????Jmeter?????????QC??????APP????????????????app?????е????????jenkins+testng+ant+webdriver??????????????JMeter????HTTP???????Selenium 2.0 WebDriver ??????