C/C++???MySQL
???????????? ???????[ 2015/11/9 11:11:34 ] ????????????? C++
???????????????C/C++????Mysql?????????api?鼮??????????????????????Linux ??C/C++?????????????????????????á?
??????????????????MySQL???????????MySQL???е?lib???????????????С????C/C++???????$MYSQL_ROOT/include???????????????·?????檔?????????У?????$MYSQL_ROOT/lib??????Link?????????-lmysqlclient???????lib??????????????????У??????????????libmysqlclient.so?????
1 ???????????????????????????
2 /*
3 * MySQLManager.h
4 *
5 * Created on: Feb 18?? 2009
6 * Author: Steven Wee
7 */
8
9 #ifndef MYSQLMANAGER_H_
10 #define MYSQLMANAGER_H_
11
12 #include "../Common/CheckStringTools.h"
13
14 #include < mysql.h>
15
16 #include < string>
17 #include < iostream>
18 #include < vector>
19
20 #include < string.h>
21
22 using namespace std;
23
24 class MySQLManager
25 {
26 public:
27 /*
28 * Init MySQL
29 * @param hosts: Host IP address
30
31 * @param userName: Login UserName
32 * @param password: Login Password
33 * @param dbName: Database Name
34 * @param port: Host listen port number
35 */
36 MySQLManager(std::string hosts?? std::string userName?? std::string password?? std::string dbName?? unsigned int port);
37 ~MySQLManager();
38 void initConnection();
39 /*
40 * Making query from database
41 * @param mysql: MySQL Object
42 * @param sql: Running SQL command
43 */
44 bool runSQLCommand(std::string sql);
45 /**
46 * Destroy MySQL object
47 * @param mysql MySQL object
48 */
49 void destroyConnection();
50 bool getConnectionStatus();
51 vector< vector< string> > getResult();
52 protected:
53 void setUserName(std::string userName);
54 void setHosts(std::string hosts);
55 void setPassword(std::string password);
56 void setDBName(std::string dbName);
57 void setPort(unsigned int port);
58 private:
59 bool IsConnected;
60 vector< vector< string> > resultList;
61 MYSQL mySQLClient;
62 unsigned int DEFAULTPORT;
63 char * HOSTS;
64 char * USERNAME;
65 char * PASSWORD;
66 char * DBNAME;
67 };
68
69 #endif /* MYSQLMANAGER_H_ */
70
71 /*
72 * MySQLManager.cpp
73 *
74 * Created on: Feb 18?? 2009
75 * Author: Steven Wee
76 */
77 #include "MySQLManager.h"
78
79 MySQLManager::MySQLManager(string hosts?? string userName?? string password?? string dbName?? unsigned int port)
80
81 {
82 IsConnected = false;
83 this ->setHosts(hosts); // ????????IP???
84 this ->setUserName(userName); // ???????????
85 this ->setPassword(password); // ??????????
86 this ->setDBName(dbName); // ???????????
87 this ->setPort(port); // ?????????
88 }
89
90 MySQLManager::~MySQLManager()
91 {
92 this ->destroyConnection();
93 }
94
95 void MySQLManager::setDBName(string dbName)
96 {
97 if ( dbName.empty() )
98 {// ????????????????
99 std::cout < < "DBName is null! Used default value: mysql" < < std::endl;
100 this ->DBNAME = new char[5];
101 strcpy(this ->DBNAME?? "mysql");
102 }
103 else
104 {
105 this ->DBNAME = new char[dbName.length()];
106 strcpy(this ->DBNAME?? dbName.c_str());
107 }
108 }
109
110 void MySQLManager::setHosts(string hosts)
111 {
112 if ( hosts.empty() )
113 {// ??????????????IP???
114 std::cout < < "Hosts is null! Used default value: localhost" < < std::endl;
115 this ->HOSTS = new char[9];
116 strcpy(this ->HOSTS?? "localhost");
117 }
118 else
119 {
120 this ->HOSTS = new char[hosts.length()];
121 strcpy(this ->HOSTS?? hosts.c_str());
122 }
123 }
124
125 void MySQLManager::setPassword(string password)
126 {// ?????????????
127 if ( password.empty() )
128 {
129
130 std::cout < < "Password is null! Used default value: " < < std::endl;
131 this ->PASSWORD = new char[1];
132 strcpy(this ->PASSWORD?? "");
133 }
134 else
135 {
136 this ->PASSWORD = new char[password.length()];
137 strcpy(this ->PASSWORD?? password.c_str());
138 }
139 }
140
141 void MySQLManager::setPort(unsigned int port)
142 {// ???????????????????????
143 if ( port )
144 {
145 std::cout < < "Port number is null! Used default value: 0" < < std::endl;
146 this ->DEFAULTPORT = 0;
147 }
148 else
149 {
150 this ->DEFAULTPORT = port;
151 }
152 }
153
154 void MySQLManager::setUserName(string userName)
155 {// ?????????????????
156 if ( userName.empty() )
157 {
158 std::cout < < "UserName is null! Used default value: root" < < std::endl;
159 this ->USERNAME = new char[4];
160 strcpy(this ->USERNAME?? "root");
161 }
162 else
163 {
164 this ->USERNAME = new char[userName.length()];
165 strcpy(this ->USERNAME?? userName.c_str());
166 }
167 }
168
169 void MySQLManager::initConnection()
170 {
171 if ( IsConnected )
172 {// ??????????????
173 std::cout < < "Is connected to server!" < < std::endl;
174 return;
175 }
176 mysql_init(&mySQLClient);// ???????????
177 if ( !mysql_real_connect( &mySQLClient?? HOSTS?? USERNAME?? PASSWORD?? DBNAME?? DEFAULTPORT?? NULL?? 0) )
178
179 {// ???????????
180 std::cout < < "Error connection to database: %s
" < < mysql_error(&mySQLClient) < < std::endl;
181 }
182 IsConnected = true;// ?????????
183 }
184
185 bool MySQLManager::runSQLCommand(string sql)
186 {
187 if ( !IsConnected )
188 {// ??????????????
189 std::cout < < "Not connect to database!" < < std::endl;
190 return false;
191 }
192 if ( sql.empty() )
193 {// SQL??????
194 std::cout < < "SQL is null!" < < std::endl;
195 return false;
196 }
197
198 MYSQL_RES *res;
199 MYSQL_ROW row;
200
201 unsigned int i??j = 0;
202
203 StringTools stringTools;
204 sql = stringTools.filterString(sql);
205
206 i = mysql_real_query(&mySQLClient??sql.c_str()??(unsigned int)strlen(sql.c_str()));// ??в??
207 if ( i )
208 {
209 std::cout < < "Error query from database: %s
" < < mysql_error(&mySQLClient) < < std::endl;
210 return false;
211 }
212 res = mysql_store_result(&mySQLClient);
213 vector< string> objectValue;
214 while( (row = mysql_fetch_row(res)) )
215 {// ?????????
216 objectValue.clear();
217 for ( j = 0 ; j < mysql_num_fields(res) ; j )
218 {
219 objectValue.push_back(row[j]);
220 }
221 this ->resultList.push_back(objectValue);
222 }
223 mysql_free_result(res); //free result after you get the result
224
225
226
227 return true;
228 }
229
230 vector< vector< string> > MySQLManager::getResult()
231 {
232 return resultList;
233 }
234
235 void MySQLManager::destroyConnection()
236 {
237 mysql_close(&mySQLClient);
238 this ->IsConnected = false;
239 }
240
241 bool MySQLManager::getConnectionStatus()
242 {
243 return IsConnected;
244 }
??????
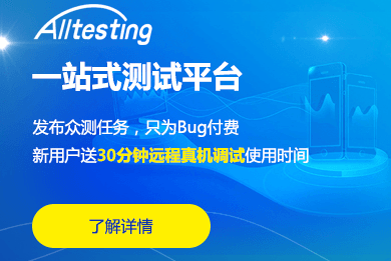
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11